In this article we will look into Prototype Design Pattern in java and try to understand what problem its solve and its practical use case. A Prototype Design Pattern is a creational pattern that helps to create new objects by copying an existing object. This pattern is particularly useful when creating objects is complex or resource-intensive.
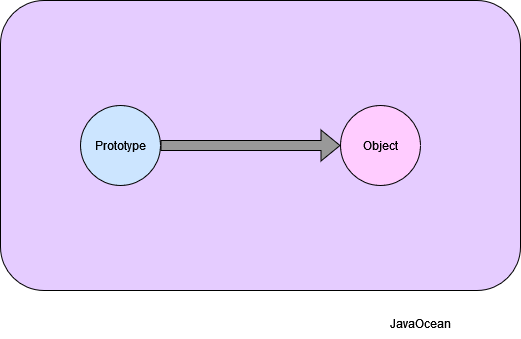
Introduction
Prototype design pattern is one of the Creational Design patterns, so it provides a mechanism of object creation by copying an existing object, known as the prototype.
This allows you to create new instances without the need to instantiate a class from scratch, which can be beneficial when the cost of object creation is high or complex.
Prototype Pattern Example
Let’s understand the prototype design pattern with an example. Suppose we have a complex Object that loads data from database, and we have requirement to modify it multiple time in program then it’s not recommended to create object using new keyword and load the data again and again from Database. Recommended ways to use the techniques of cloning existing object and modify its data as per needed.
Prototype design pattern mandates that the Object which you are copying should provide the copying feature. It should not be done by any other class. As per the requirement we can use any of copying techniques weather its it shallow or deep copy of the Object properties.
package com.javaocean.design.prototype; import java.util.ArrayList; import java.util.List; public class Student implements Cloneable{ private List<String> stuList; public Student(){ stuList = new ArrayList<String>(); } public Student(List<String> list){ this.stuList=list; } public void loadData(){ //read all Student from database and put into the list stuList.add("Ajay"); stuList.add("Raj"); stuList.add("Rohit"); stuList.add("John"); } public List<String> getStuList() { return stuList; } @Override public Object clone() throws CloneNotSupportedException{ List<String> temp = new ArrayList<String>(); for(String s : this.getStuList()){ temp.add(s); } return new Student(temp); } }
Lets Test the Prototype design pattern example
package com.javaocean.design.test; import java.util.List; import com.javaocean.design.prototype.Student; public class PrototypePatternTest { public static void main(String[] args) throws CloneNotSupportedException { Student student = new Student(); student.loadData(); //Use the clone method to get the Student object Student studentNew = (Student) student.clone(); Student studentNew1 = (Student) student.clone(); List<String> list = studentNew.getStuList(); list.add("John"); List<String> list1 = studentNew1.getStuList(); list1.remove("David"); System.out.println("Student List: "+student.getStuList()); System.out.println("StudentNew List: "+list); System.out.println("StudentNew1 List: "+list1); } }
Prototype Design Pattern Advantages
- Object Cloning: The pattern allows you to create new objects by copying existing ones, which promotes code readability. This is especially beneficial when objects have complex or resource-intensive initialization processes.
- Reduced Creation Overhead: As objects are created by cloned methodology , it can significantly reduce the overhead associated with expensive object initialization and very beneficial in nature.
- Customization: This provide flexibility in object creation. It can be easily customized to suit specific requirements while retaining the common characteristics of the prototype.
- Object Creation Logic: The Pattern abstracts the complex object creation logic, that help to maintain cleaner and more readable code.
- Streamlined Object Creation: The pattern provides a structured and consistent way to create objects, making the codebase more organized and easier to maintain.
Prototype Design Pattern Disadvantages
- Shallow vs Deep Copy: In scenarios where objects contain references to other objects (e.g., nested objects), cloning might affect the original object and vice versa. Deep copying may be required, which can be complex to implement.
- Object State Managing : If an object has an internal state that should not be shared across clones, careful management of the object state is necessary to ensure that each clone maintains its integrity.
- Creating Concrete Prototypes: This pattern involves creating concrete prototype classes and customizing their clone methods. This can introduce additional classes and complexity .
- Limited Applicability: The Prototype Pattern is only suitable when the cost of creating objects from scratch is high. other wise pattern may introduce unnecessary complexity.
- Compatibility with Serialization: If you need to clone objects that are serializable, you might encounter challenges related to object serialization and deserialization.
You may find other post on different topic that might be helpful, Please have a look