In this article we will look in to the new feature called method reference in Java and explore the different types of method references in details with example.
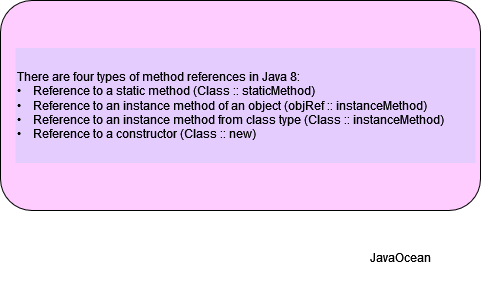
Introduction
Method reference is a special type of lambda expression which are often used to creates simple lambda expressions by referring existing method. Method references can be used to simplify the code and make it more readable.
Method references can be used to simplify the code and make it more readable; we’ll explore the different types of method references in Java 8 with examples.
There are four types of method references in Java 8:
- Reference to a static method (Class :: staticMethod)
- Reference to an instance method of an object (objRef :: instanceMethod)
- Reference to an instance method from class type (Class :: instanceMethod)
- Reference to a constructor (Class :: new)
Method References in java 8
Java has introduced a new operator:: (double colon) called as Method reference delimiter. Let’s explore each of these types in detail:
- Reference to a static method: A reference to a static method can be created using the syntax ClassName::staticName. For example:
public class MyUtils { public static int multiply(int a, int b) { return a * b; } } public class Main { public static void main(String[] args) { IntBinaryOperator operator = MyUtils::multiply; int result = operator.applyAsInt(6, 5); System.out.println(result); } }
In above example,
- Here we are referring to the static method multiply of MyUtils class using the MyUtils::multiply
- And then assigned this reference to an IntBinaryOperator Which is functional interface and takes two integers as parameters and returns an integer value.
- We called the applyAsInt method on this variable with the arguments 4 and 5 to get the result, which is 30.
- Reference to an instance method of an object: A reference to an instance method of an object can be created using the syntax object::methodName. For example:
public class Employee { private String name; public Employee(String name) { this.name = name; } //an instance method public String getName() { return name; } } public class Main { public static void main(String[] args) { Employee employee = new Employee ("JavaOcean"); Supplier<String> supplier = employee::getName; String name = supplier.get(); System.out.println(name); } }
In above example,
- Here we created a reference to the getName() instance method of the I.e. employee::getName
- We called the get method on this variable to get the name of the Employee, which is “JavaOcean”.
- Reference to an instance method of a particular type: A reference to an instance method of an arbitrary object of a particular type can be created using the syntax ClassName::methodName. For example:
public class MyUtils { public boolean startsWithUppercase(String s) { return Character.isUpperCase(s.charAt(0)); } } public class Main { public static void main(String[] args) { Predicate<String> predicate = MyUtils::startsWithUppercase; boolean result = predicate.test("javaocean"); System.out.println(result); } }
In this example,
- Here we are referring to the startsWithUppercase method of the MyUtils class using the MyUtils::startsWithUppercase and assigned it to predicate.
- Then we called the test method on this predicate variable with the argument “javaocean” to get the result, which is true.
- Reference to a constructor: A reference to a constructor can be created using the syntax ClassName::new. For example:
public class Product { private String name; private int price; public Product(String name, int price) { this.name = name; this.price = price; } // Getter and setter methods } public class Main { public static void main(String[] args) { BiFunction<String, Integer, Product> productConstructor = Product::new; Product product = productConstructor.apply("Apple Iphone", 1500); System.out.println(product.getName()); System.out.println(product.getPrice()); } }
In this example
- Here we created a reference to the Product constructor by using the Product::new and assigned to Bifunction function.
- Then we called the apply method on this variable by passing value as “Apple Iphone ” and 1500 to create a new Product
Few other example of constructor reference are
- String::new;
- Integer::new;
- ArrayList::new;
- CustomClass::new
You may find other post on different topic that might be helpful, Please have a look