In this tutorial, we will try to compare the two main ways of initializing arrays Array.asList() and List.of() and also discuss the Difference between.
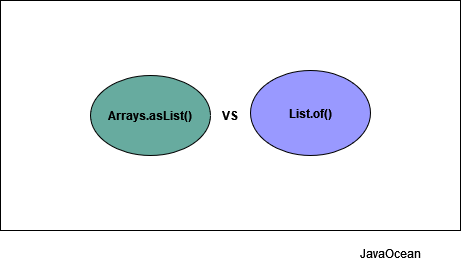
Introduction
Java provides several convenient ways for creating lists, like Arrays.asList() and List.of(), both are need to create a small list or convert an array into a list for convenience.
Arrays is a utility class present in java.util package and has been there since Java version 1.2 whereas List.of() is introduce in Java 9.
Both methods are frequently use to create list easily, but both exbibit some different properties, we should aware before using these, so let’s understand it in details and try to understand the case when to uses and how they are different.
Arrays.asList()
Arrays.asList() is a method introduce in java since the 1. 2 versions of Java and provides a convenient way to create a fixed-size list backed by the specified array. It can take an array as input and create the List object of the provided array:
List<Integer> mutableList = Arrays.asList(1, 2, 3, 4);
Unsupported exception on the Returned List
The method asList() returns a fixed-size list. Therefore, when we perform any adding and removing new elements will throws an UnsupportedOperationException
package com.javaocean; import java.util.Arrays; import java.util.List; public class AsList { public static void main(String[] args) { Integer[] array = new Integer[] { 1, 2, 3, 4 }; List<Integer> mutableList = Arrays.asList(array); mutableList.add(5); // Exception in thread "main" java.lang.UnsupportedOperationException mutableList.remove(0); // UnsupportedOperationException System.out.println(mutableList); } }
Working With Arrays
list doesn’t create a copy of the input array. Instead, it wraps the original array with the List interface. Therefore, changes to the array reflect on the list too.
package com.javaocean; import java.util.Arrays; import java.util.List; public class AsList { public static void main(String[] args) { Integer[] array = new Integer[] { 1, 2, 3, 4 }; List<Integer> mutableList = Arrays.asList(array); array[0] = 12; // print both array and list for (int i = 0; i < array.length; i++) { System.out.print("ArrysValue :" +array[i]); } // printing List value System.out.println(" List Value : " + mutableList); } }
//Output: 12 2 3 4 List : [12, 2, 3, 4]
Modifying the value of Returned List
List returned by Arrays.asList() is mutable. That is, we can change the individual elements of the list
List<Integer> mutableList = Arrays.asList(1, 2, 3, 4); mutableList.set(1, 1000);
Change on the list will also reflect on arrays.
Integer[] mutableList = new Integer[]{1, 2, 3}; List<Integer> list = Arrays.asList(mutableList);list.set(0,1000);
Please make a note that the Arrays.asList() method returns a fixed-size list. If you want to get a sizable collection, construct an ArrayList object that wraps the list returned by Arrays.asList() method.
In other word , if you want to initialize a List with some values in a single line, and later you can add/remove elements if needed. Then you should construct an ArrayList like this:
List<String> listFruits = new ArrayList<>(Arrays.asList("Apple", "Coconut", "Banana", "Guava", "Mango"));
Using List.of()
In contrast to Arrays.asList(), Java 9 introduced a more convenient method, List.of(). This creates instances of unmodifiable List objects
package com.javaocean; import java.util.List; public class ListOfJava { public static void main(String[] args) { Integer[] array = new Integer[]{1,2,3,4}; List<Integer> immutableList = List.of(array); System.out.println(immutableList); } }
List.of() returns an immutable list that is a copy of the provided input array. The main difference from Arrays.asList() is that, changes to the original array aren’t reflected on the returned list:
package com.javaocean; import java.util.List; public class ListOfJava { public static void main(String[] args) { Integer[] array = new Integer[] { 1, 2, 3, 4 }; List<Integer> immutableList = List.of(array); array[0] = 18; // Only reflect on arrays not on list // print both array for (int i = 0; i < array.length; i++) { System.out.println("ArrysValue :" + array[i]); } //print List System.out.println(immutableList); } }
Modifying list throw UnsupportedOperationException
As List.of() returns an immutable list, if we try to modify the element of the list it will throw UnsupportedOperationException
package com.javaocean; import java.util.List; public class ListOfJava { public static void main(String[] args) { Integer[] array = new Integer[] { 1, 2, 3, 4 }; List<Integer> immutableList = List.of(array); immutableList.set(0, 20); // UnsupportedOperationException System.out.println(immutableList); } }
Null Values
List.of() doesn’t allow null values as input and will throw a NullPointerException
Example List.of(1, null, 2);
Difference between Arrays.asList() and List.of()
Lets understand the differences between Arrays.asList() and List.of(), let’s explore their respective use cases:
Arrays.asList()
- Modifiability: The resulting list from Arrays.asList() is modifiable, allowing you to only update its elements and not its structure (that is add/remove not allowed).
- Backed by an array: The list is backed by the original array, so any changes to elements of the list will affect the underlying array and vice versa.
- Fixed-size limitation: Although modifiable, the size of the list returned by Arrays.asList() is fixed, preventing structural modifications such as adding or removing elements.
- Null values: Unlike List.of(), Arrays.asList() allows null elements.
List.of()
List.of() is a factory method introduced in Java 9 that creates an immutable list containing the specified elements. Few key points to not about this are mention below;
- Immutability: As it returns list as immutable, it meaning we cannot be modified after creation.
- Fixed-size: The list created by List.of() has a fixed size and does not support adding or removing elements.
- Null values: List.of() does not allow null elements, it will throw a NullPointerException
You may find other post on different topic that might be helpful, Please have a look